Most Common API Terminology: Ultimate Guide for Beginners
APIs have become essential for allowing different software applications to "talk" to each other and share data. However, all the technical jargon around APIs can be confusing for beginners. This guide will explain API terminology in simple, easy-to-understand language.
By the end, you'll be fluent in API basics like:
What an API actually is and how they enable connectivity
The most common architectural style for APIs (REST)
Key API components like endpoints, requests, responses
Crucial API concepts for security, performance, and scalability
P.S., looking for a trusted source for discovering and integrating new APIs? Check out API League's user-friendly directory. Our vast API glossary is a treasure trove of APIs, SDKs, and dev tools to supercharge your workflow.
So, Let's get started!
What is an API?
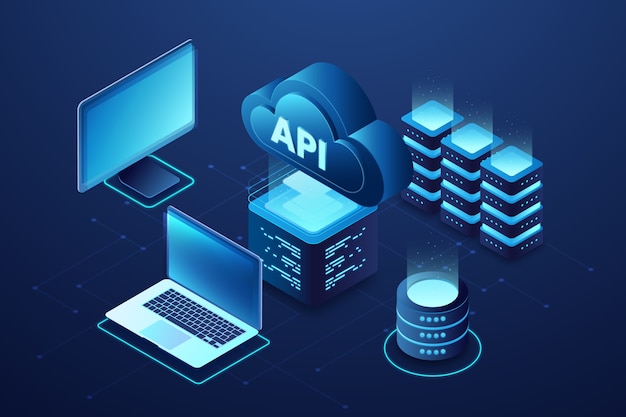
An API (Application Programming Interface) is a set of rules, protocols, and standards that govern how different software applications communicate and exchange data with each other.
APIs act as intermediaries or messengers, enabling different systems and applications to interact seamlessly, even if they are built using different programming languages or technologies.
APIs define the methods, data formats, and conventions that applications must follow to request and exchange information effectively.
Think of an API as a waiter in a restaurant: it takes your order (request) from you, relays it to the kitchen (backend system), and brings back the prepared dish (response) to you.
For example, API League's directory simplifies the often daunting task of finding the right APIs for your project's needs. With a vast collection at your fingertips, you can easily discover and integrate powerful interfaces into client applications.
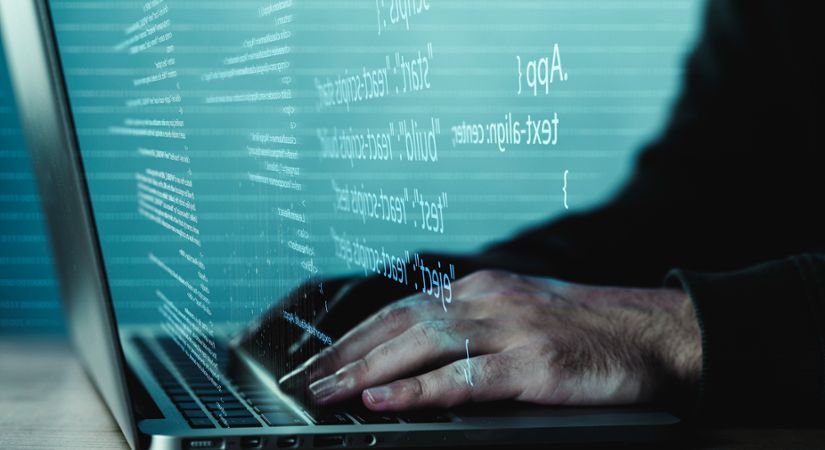
Why Are APIs Important?
APIs enable different software systems and applications to communicate and share data seamlessly, fostering integration and interoperability.
API Gateway provides a standardized and controlled way to access and leverage data and functionality from third-party services, platforms, or databases.
APIs facilitate innovation by allowing developers to build new applications and services on top of existing systems and data sources.
They enable organizations to expose their data and functionality to partners, customers, or the public in a secure and controlled manner.
The Most Popular Type of API: REST
REST (Representational State Transfer) is the most widely adopted architectural style for building APIs in today's web-driven world.
RESTful APIs leverage standard HTTP methods (Hypertext Transfer Protocol) like GET, POST, PUT, and DELETE to perform operations on "resources" (pieces of data) hosted on servers.
This approach aligns seamlessly with how the web operates, making REST APIs a natural choice for building robust, scalable, and lightweight web services.
Key Advantages of RESTful APIs
Simplicity: RESTful APIs use straightforward URLs and HTTP methods, making them easy to understand and implement.
Statelessness: Each request from a client to the server contains all the necessary information, eliminating the need for the server to maintain the session state.
Scalability: RESTful APIs are highly scalable, as they are designed to handle large volumes of traffic and can be easily load-balanced across multiple servers for web services.
Cacheability: Responses from RESTful APIs can be cached, improving performance and reducing server load.
Flexibility: RESTful APIs support various data formats, such as JSON, XML, and plain text, enabling versatile data exchange.
API League's platform simplifies the process of discovering, understanding, and integrating RESTful APIs for developers. With its extensive directory of APIs, practical examples, and detailed documentation, API League enables you to leverage the power of RESTful APIs effectively.
Navigating Key API Terminology

As a beginner in the world of APIs, understanding the terminology can feel like learning a new language. However, with the right guidance, you'll soon be fluent in the language of APIs. This section aims to demystify the most critical API terms you'll encounter, ensuring you have a solid foundation to build upon.
Endpoint
An API endpoint is a unique URL that represents a specific resource or collection of resources within an API.
Endpoints are the entry points where clients can interact with and perform operations on data via the API.
Endpoints typically follow a structured URL pattern that includes the API's base URL, version, and resource paths. Example: https://api.example.com/v1/products (represents a collection of product resources for version 1 of an e-commerce API)
Best Practices for Endpoints:
Thorough Documentation: Documenting your endpoints, including URL patterns, HTTP methods, request/response formats, and required parameters, enhances developer experience and streamlines integration. Tools like Swagger or Postman can auto-generate documentation from your API specification.
Naming Conventions: Using plural nouns for endpoint paths representing collections (e.g., /users) and singular nouns for individual resources (e.g., /users/34) is a common best practice.
Versioning: Including the API version in the endpoint URL (e.g., /v1/products) allows for better control and management of API changes.
Request
A request is a message sent by the client to trigger an API call and initiate server processing.
It includes the HTTP method (GET, POST, PUT, DELETE, etc.), headers, and message body (if applicable).
Requests contain all the necessary information for the server to understand and process the API call.
Best Practices for API Requests:
Proper formatting: Ensure requests are formatted correctly based on the API's documentation (e.g., JSON, XML, or form data).
Authentication: Include appropriate authentication credentials (API keys, tokens, etc.) in the request headers or body.
Parameter handling: Properly handle and validate any required or optional parameters in the request.
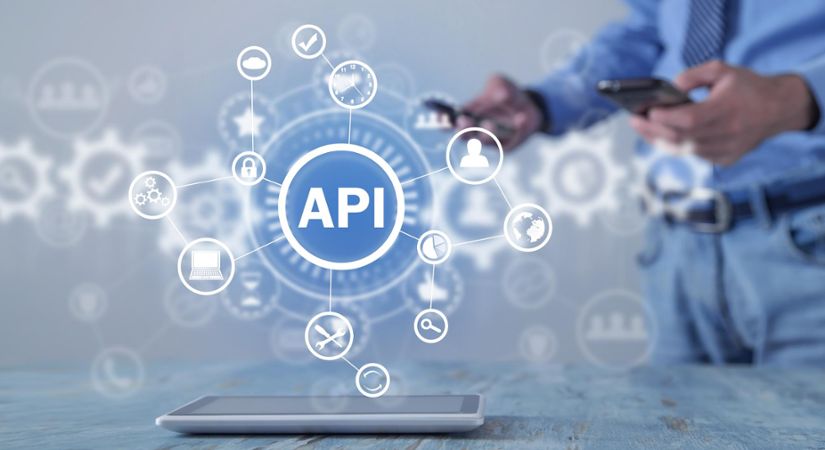
Response
The response is the data returned from the server to the client after processing an API request.
It contains HTTP status codes, response headers, and the payload (data) in the response body.
Responses provide crucial information about the success or failure of the API call.
Best Practices for API Responses:
Clear error messages: Well-designed APIs should return responses with clear and descriptive error messages, improving the developer experience when issues arise.
Consistent formatting: Ensure responses are consistently formatted based on the API's documentation (e.g., JSON, XML).
Caching headers: Include appropriate caching headers in responses to optimize performance and reduce server load.
HTTP Methods
HTTP methods (or verbs) define the type of operation that should be performed on a given resource in an API.
The most common HTTP methods used in APIs are:
GET: Retrieve existing data
POST: Create new data
PUT: Update existing data
DELETE: Remove data
Example: In a blog API, a GET request to /posts might fetch all published posts, while a POST request to the same endpoint would create a new blog entry.
RESTful Constraint: A core principle of RESTful APIs is that the HTTP method implies the operation. Using the correct method for the intended operation (GET for retrieval, POST for creation, etc.) is essential for maintaining RESTful API design.
Headers
Headers provide crucial metadata for both requests and responses in API communication.
Common headers include:
Content-Type: Specifies the format of the data (e.g., JSON, XML)
Authorization: Carries authentication credentials or tokens
Cache-Control: Provides caching instructions for responses
User-Agent: Identifies the client application making the request
Well-designed APIs should return descriptive headers to enhance the developer experience and provide valuable information about the request/response.
A fintech startup used API League's header inspection tools to analyze responses from a payment gateway API. This allowed them to properly handle caching and identify suspicious traffic sources.
Status Codes
Status codes are numeric codes included in API responses to indicate whether the request was successful, failed due to a client error, or encountered a server error.
Common status code categories:
100s: Informational codes
200s: Success codes (e.g., 200 OK)
300s: Redirection codes
400s: Client error codes (e.g., 404 Not Found, 401 Unauthorized)
500s: Server error codes (e.g., 500 Internal Server Error)
Payload/Body
Every API request and response carries information, just like a delivery truck carries packages. This data is called the payload or body.
The payload can be formatted in different ways, like JSON, XML, or even form data, depending on the API's instructions. Think of it like different types of boxes the delivery truck can carry.
JSON (JavaScript Object Notation) is the most popular format these days. It's lightweight, easy to understand, and human-readable, kind of like clear labeling on a package! This makes it perfect for sending and receiving data efficiently.
Query Parameters
Query parameters are like filters you can add to your API requests to get specific data. They are optional parameters appended to the API endpoint URL.
They allow clients to filter, sort, search, or paginate the response data according to their needs.
For instance, the URL /products?category=electronics&sort=price_desc would request a list of electronic products, sorted by price from highest to lowest. Pretty cool, right? With query parameters, you can fine-tune your API requests to get exactly the data you need.
Resources
Resources are the data entities or objects that an API exposes and acts upon via its endpoints (e.g., /users, /products).
Resources model real-world concepts and serve as the building blocks of an API's functionality.
Nested Resources: APIs can expose nested resource structures like /companies/34/employees to logically organize access to related data entities.
Collections
Collections refer to groups of similar resources treated as a single unit by the API (e.g., /users is a collection of individual user resources).
Well-designed APIs provide parameters to filter, sort, search, and paginate large collections for better usability and performance.
Idempotent
An API operation is considered idempotent if it has the same effect whether called once or multiple times with the same input parameters.
Examples of idempotent operations: PUT (update) and DELETE requests.
Benefits of Idempotency: It helps prevent unintended side effects from duplicate API requests, improving reliability and developer experience.
Stateless
Statelessness is a key constraint in RESTful APIs, where no client context or state is stored between requests.
Each request from a client to the API must contain all the necessary information to be processed independently.
Use Stateless Design for Public APIs, services with high scalability needs, and multi-device/client access scenarios.
GraphQL
GraphQL is an open-source data query and manipulation language that provides a flexible alternative to traditional REST APIs.
Industry giants like Facebook, GitHub, Shopify, and PayPal have embraced GraphQL for its ability to fetch only the needed data, reducing over-fetching and under-fetching issues.
GraphQL allows clients to request and receive exactly the data they need, improving performance and reducing bandwidth usage.
Authentication
Authentication is the process of verifying a client's identity before granting access to an API.
Common authentication methods include API keys, OAuth, and OpenID Connect.
Foundational Security: Robust authentication is the first line of defense against threats like credential stuffing, man-in-the-middle attacks, and data breaches targeting APIs.
Authorization
Authorization refers to the process of determining what resources and operations a client is permitted to access or perform within an API.
Common authorization methods include:
API Keys: Secret keys issued for identification and access control.
OAuth: Open standard for secure, delegated access to resources.
JWT (JSON Web Tokens): Self-contained, signed tokens transmitting data securely.
API Security Risks: A recent survey revealed that 92% of organizations faced an API security incident last year, highlighting the importance of robust authentication and authorization mechanisms.
API League's authentication tools simplify securing your APIs against threats like credential stuffing or man-in-the-middle attacks.
Delegated Authorization with OAuth:: OAuth enables delegated authorization, allowing users to grant limited access to their data without sharing account credentials.
Web API
Imagine you're building a weather app. Weather data is constantly changing, and you don't want to manually update your app every time. That's where web APIs come in! They act as middlemen, allowing your app to talk to weather services and retrieve the latest information seamlessly.
Web APIs use HTTP, the same protocol that lets you browse websites, to send requests and receive data. Think of it like a universal language for machines to communicate.
The importance of web APIs is undeniable. Studies show that API traffic was recently estimated to account for 80% of all internet traffic! They're the backbone of modern web and mobile applications, powering everything from social media feeds to real-time news updates.
SOAP API
SOAP (Simple Object Access Protocol) is an older protocol kind of like the fax machine of the API world. It uses XML (Extensible Markup Language), a complex markup language, to send messages and data between applications.
While SOAP is less common for new projects these days, it's still used in some critical enterprise systems like banking or finance, where updating everything to newer protocols might be too complex or expensive.
So, while SOAP knowledge might not be essential for every aspiring developer, it can be a valuable skill for those interested in niche career opportunities working with legacy systems. You'll be the decoder ring for these older technologies!
API Keys
API keys are unique secure tokens used to identify and authenticate clients connecting to an API.
An API key acts like a secret handshake or membership card. It identifies you (or your application) to the API and grants you access to its data or functionalities.
These keys are unique strings of characters that should be kept confidential. Never store them directly in your code! Instead, use secure methods like environment variables or hashed storage to keep them safe from prying eyes. Think of it like keeping your membership card locked away in a safe.
JWT (JSON Web Token)
JWT (JSON Web Token) is an open standard for securely transmitting data as JSON objects between parties in a web application scenario.
JWT Structure: A JWT consists of a Header (containing type and algorithm metadata), a Payload (containing claims and data), and a Signature (ensuring integrity).
Rate Limiting
Rate limiting is the practice of restricting how frequently clients can call an API within a specific time window to prevent abuse and balance server load.
Throttling Policies: Rate limiting can be applied at the IP address or authenticated user level while allowing unlimited access to health check endpoints.
Pagination
Pagination is the process of splitting large response datasets into smaller chunks, allowing clients to retrieve data via multiple API requests.
Pagination Metadata: Well-designed APIs include metadata such as total record count and links to next/previous pages for seamless client-side iteration.
Caching
Caching involves temporarily storing API response data to improve performance by serving subsequent requests from the cached copy instead of the source.
Cache Busting: Versioning APIs, leveraging cache-control headers, and using a Content Delivery Network (CDN) can help prevent serving stale cached data.
CORS (Cross-Origin Resource Sharing)
CORS (Cross-Origin Resource Sharing) is a security mechanism that controls how web browsers allow or restrict cross-origin API requests from executing.
CORS in Action: Enabling CORS policies is critical for browser-based applications to access APIs hosted on different domains.
Versioning
Versioning is the practice of creating different versions of an API to introduce breaking changes without disrupting existing client integrations.
Versioning Approaches: Common approaches include URI versioning (e.g., /v1/resources), query parameter versioning (e.g., ?version=2), custom headers (e.g., Accept: version=3), and content negotiation (via Accept types).
Webhook
A webhook is a way for APIs to provide real-time data by pushing notifications to a specified URL when certain events occur.
Bi-directional Communication: While APIs use a request-response model, webhooks enable event-driven, server-to-client communication.
Mocking
Mocking involves simulating API behavior by creating a mock service with sample responses to test API integration before the real implementation.
Mocking Benefits: Enables parallel API development, decoupling client and server work, and avoids spinning up dependencies during testing.
Sandboxing
A sandbox is a safe, isolated test environment that allows developers to explore and test an API's functionality before integration.
API sandboxes provide mock data, pre-populated test accounts, and a space to experiment without impacting production systems.
Tool Recommendation: API League integrates with leading sandbox providers to streamline creating, sharing, and managing API sandboxes for your teams.
OpenAPI Specification (Swagger)
The OpenAPI Specification (formerly known as Swagger) is a standard, programming language-agnostic specification for describing REST APIs in a structured, machine-readable format.
Power of Open Standards: By adopting the OpenAPI specification, your API documentation becomes readable by a vast ecosystem of tools for code generation, testing, discovery, and more.
Swagger in Action: API League leverages the OpenAPI specification to auto-generate crisp, interactive documentation, simplifying the onboarding process for developers integrating your APIs.
Grasping these core API concepts and terms equips you to navigate this powerful interconnected API ecosystem with confidence. If jargon ever has you stumped, revisit this guide as your personal translator!
Ready to Put Your API Knowledge Into Practice?
Talk the API talk with confidence and test out your knowledge of APIs. Understanding these core API concepts and terms helps you to navigate this powerful ecosystem with confidence. As you move forward in your journey, you'll encounter more nuanced terminology - but this guide ensures you've got a rock-solid foundation.
Key Takeaways
APIs are the backbone enabling different apps and systems to communicate
REST is the dominant architectural style, leveraging HTTP verbs
Implementing strong API security via authentication is crucial
Proper documentation enhances the developer experience
Integration and scaling of a software development lifecycle require mindful rate limiting and pagination
Make the process easier with API League! Get access to our vast directory of APIs with detailed documentation and examples. Find the perfect APIs for your projects and integrate them seamlessly into your workflow. Our intuitive platform empowers you throughout the entire API lifecycle - from discovery software development to deployment and beyond.
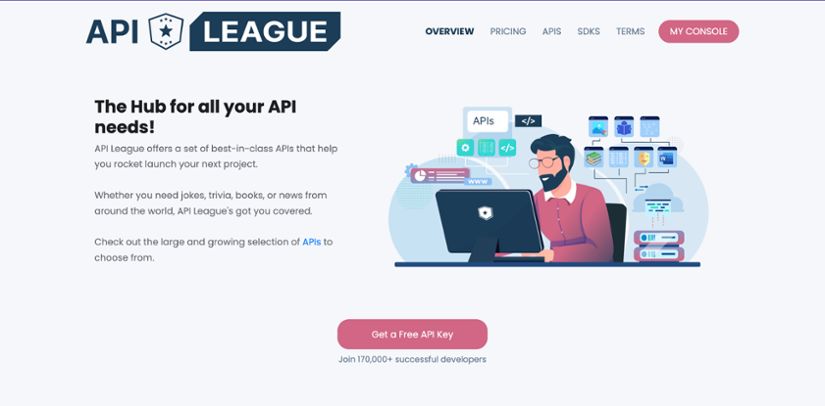